The difference between get and filter methods in Django
Get is to obtain an object, and sometimes the situation of DoesNotExist: User matching query does not exist occurs.
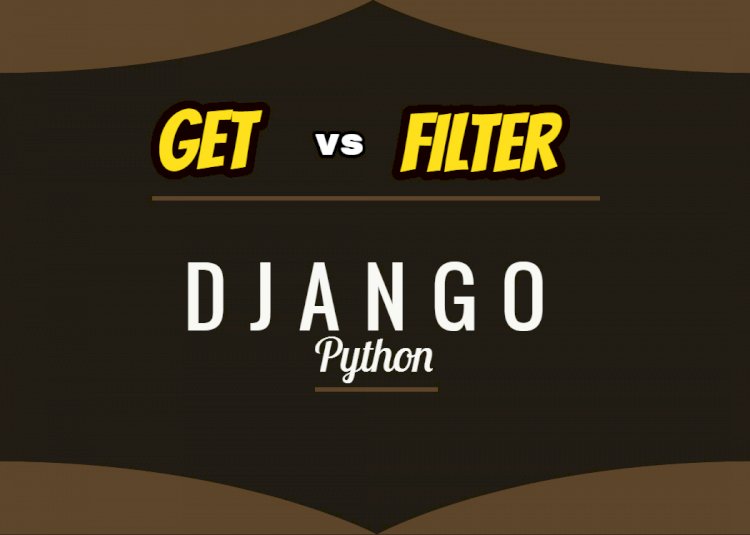
get
Get is to obtain an object, and sometimes the situation of DoesNotExist: User matching query does not exist occurs.
One of the reasons I encountered is: get failed to get the content because there is no data in the User table in the database.
Solution: Fill in the relevant data in the User table, and then use the following code.
# solution one: get
profile_mail = User.objects.get(uid=uid)
print(profile_mail)
if not profile_mail:
return False
print(profile_mail.mail)
return JsonResponse(profile_mail.mail, safe=False)
What profile_mail gets is an object Object. To get mail, you need to use .mail.
The output content is as follows:
User object (11) 123@qq.com
There is also a mistake here: In order to allow non-dict objects to be serialized set the safe parameter to False.
Because the return is Json data, it needs to be serialized, so return JsonResponse(profile_mail.mail, safe=False) should add a safe=False.
What get returns is an object, only one can be returned, if the record does not exist, it will report an error.
filter
When faced with multiple objects, you can't use get, but filter.
Solution:
# solution two: post
profile_mail = User.objects.filter(uid=uid)
print(profile_mail)
for i in profile_mail:
print(i.mail)
return JsonResponse(i.mail, safe=False)
For the content obtained, filter returns a list of objects. If the record does not exist, it returns [].
The output content is as follows:
<QuerySet [<User: User object (11)>]> 123@qq.com
Filter values and values_list
values(*fields)
Returns a ValuesQuerySet (a subclass of QuerySet), and returns a dictionary during iteration, representing an object, but not a model instance object.
profile_mail = User.objects.filter(uid=uid)
print(profile_mail)
profile_mail = User.objects.filter(uid=uid).values()
print(profile_mail)
The output content is as follows:
<QuerySet [<User: User object (11)>]>
<QuerySet [{'uid': 11, 'user_name': 'asdsa222', 'user_image': '', 'password': 'e10adc3949ba59abbe56e057f20f883e', 'mail': '123@qq.com', 'authority': 0}]>
values() receives the optional positional parameter *fields, which specifies which fields the SELECT should restrict. For example, filter mail information as follows:
profile_mail = User.objects.filter(uid=uid).values('mail')
print(profile_mail)
The output content is as follows:
<QuerySet [{'mail': '123@qq.com'}]>
values_list(*fields, flat=False)
What is returned is a tuple instead of a dictionary. Each tuple contains the value of the field passed to the values_list() call, so the first element is the first field, and so on.
profile_mail = User.objects.filter(uid=uid).values_list('uid','mail')
print(profile_mail)
The output content is as follows:
<QuerySet [(11, '123@qq.com')]>
If only one field is passed, you can also pass the flat parameter. If True, it means that the returned result is a single value instead of a tuple.
profile_mail = User.objects.filter(uid=uid).values_list('mail', flat=True)
print(profile_mail)
The output content is as follows:
<QuerySet ['123@qq.com']>
What's Your Reaction?
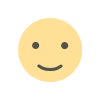
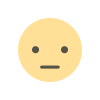




