Python Technical Interviews - 5 Things You MUST KNOW
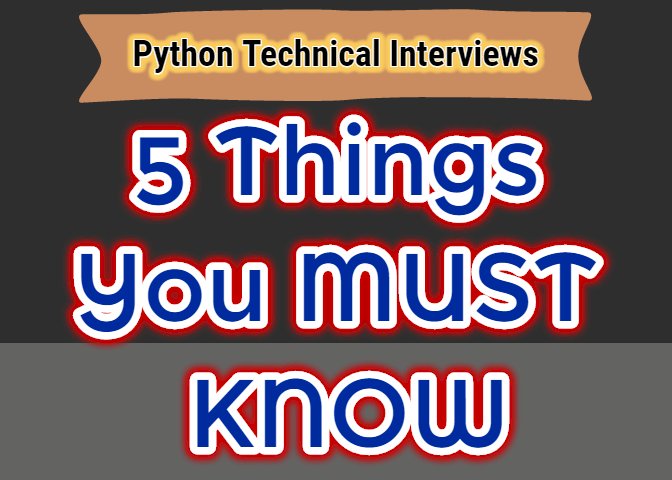
Python is a popular programming language that is frequently used in technical interviews. To excel in Python technical interviews, there are several essential concepts and techniques you should be familiar with. In this article, we will discuss five important things you must know about Python to enhance your chances of success in technical interviews. We will cover topics such as object-oriented programming, string formatting, data structures, leveraging the Python standard library, and utilizing list comprehension. Let's dive in!
1. Object-Oriented Programming in Python
During a technical interview, you may encounter questions related to object-oriented programming (OOP) in Python. It is important to understand the basics of creating classes and objects in Python. Here's an example of how to create a class for employees in a company:
By utilizing the __init__
function, you can initialize class variables such as first_name
, last_name
, salary
, and even generate the email address based on these variables. Additionally, you can define other methods specific to the employee class, such as set_salary
, to update the salary attribute.
2. Inheritance in Python
Inheritance allows you to create subclasses that inherit attributes and methods from their parent classes. Let's say we want to create a subclass called Developer
that inherits from the Employee
class:
In this example, the Developer
class inherits the attributes and methods of the Employee
class by utilizing the super()
function. Additionally, the Developer
class introduces a new attribute called programming_languages
and a method called add_language
, which allows adding new programming languages to the developer's skillset.
3. String Formatting in Python
Properly formatting strings is crucial for presenting data effectively. In Python, there are different approaches to string formatting. One commonly used method is the %
operator. Here's an example:
The %s
and %d
are placeholders that get substituted with the values of name
and age
, respectively. This allows for dynamic and formatted string output.
In Python 3.6 and above, formatted string literals or f-strings provide a more concise and readable approach to string formatting:
Using the f
prefix, you can directly embed variables within the string by enclosing them in curly braces {}
. This simplifies the process of string formatting.
4. Data Structures in Python
Understanding different data structures in Python is essential for optimizing your algorithms. Here are some commonly used built-in data structures and their functionalities:
-
Lists: Lists are ordered sequences that can store elements of varying types. They provide flexibility and come with built-in functions like
append
,pop
,insert
,sort
, andremove
. Lists can also mimic other data structures like queues and stacks. -
Sets: Sets are iterable data structures that only contain unique values. They offer useful methods such as
add
,remove
,pop
,union
, andintersection
. Sets are commonly used to find unique solutions or perform intersection/union operations. -
Dictionaries: Dictionaries enable mapping keys to values. They are efficient for quick lookup operations (O(1)). Dictionaries are often used when a problem requires mapping or storing key-value pairs.
5. Leveraging the Python Standard Library
Python's extensive standard library provides numerous pre-built modules and functions that can simplify complex tasks. During a technical interview, showcasing your knowledge of the standard library can be advantageous. Here are a couple of examples:
-
Collections module: The collections module offers useful data structures beyond the built-in ones. For instance,
defaultdict
provides a default value for unset keys, andCounter
allows efficient counting of hashable objects. -
String formatting and manipulation: Python's standard library includes modules such as
string
andre
(regular expressions) that offer powerful tools for string manipulation and formatting.
In Python technical interviews, demonstrating proficiency in object-oriented programming, string formatting, data structures, leveraging the standard library, and utilizing list comprehension can significantly enhance your chances of success. By understanding these core concepts and techniques, you will be well-prepared to tackle coding problems and showcase your Python skills effectively. Remember to practice and familiarize yourself with these concepts to ace your next technical interview. Good luck!
What's Your Reaction?
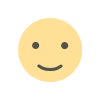
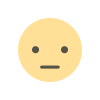




