24 JavaScript loop traversal methods
Today we look at the basics and look at those loop traversal methods in [removed]

1. Array traversal method
1. forEach()
forEach
The method is used to call each element of the array and pass the element to the callback function. The callback function is called for each value in the array. The syntax is as follows:
array.forEach(function(currentValue, index, arr), thisValue)
The first parameter of this method is the callback function, which must be passed. It has three parameters:
- currentValue: required. Current element
- index: optional. The index value of the current element.
- arr: Optional. The array object to which the current element belongs
let arr = [1,2,3,4,5]
arr.forEach((item, index, arr) => {
console.log(index+":"+item)
})
This method can also have a second parameter, which is used to bind the internal this variable of the callback function (provided that the callback function cannot be an arrow function, because the arrow function does not have this):
let arr = [1,2,3,4,5]
let arr1 = [9,8,7,6,5]
arr.forEach(function(item, index, arr){
console.log(this[index]) // 9 8 7 6 5
}, arr1)
Notice:
- The forEach method does not change the original array, nor does it return a value;
- ForEach cannot use break and continue to jump out of the loop. When using return, the effect is the same as using continue in a for loop;
- The forEach method cannot traverse objects, it is only suitable for array traversal.
2. map()
map()
The method returns a new array, and the elements in the array are the values processed by the original array elements after calling the function. This method processes the elements sequentially in the order of the original array elements. The syntax is as follows:
array.map(function(currentValue,index,arr), thisValue)
The first parameter of this method is the callback function, which must be passed. It has three parameters:
- currentValue: Required. The value of the current element;
- index: optional. The index value of the current element;
- arr: Optional. The array object to which the current element belongs.
let arr = [1, 2, 3];
arr.map(item => {
return item + 1;
})
// Output result: [2, 3, 4]
The second parameter of this method is used to bind the this variable inside the parameter function, which is optional:
let arr = ['a', 'b', 'c'];
[1, 2].map(function (e) {
return this[e];
}, arr)
// Output result: ['b', 'c']
This method can also be chained:
let arr = [1, 2, 3];
arr.map(item => item + 1).map(item => item + 1)
// Output result: [3, 4, 5]
Notice:
- The map method will not detect empty arrays;
- When the map method traverses the array, it will return a new array without changing the original array ;
- The map method has a return value, which can be returned. The return value is supported in the callback function of the map;
- The map method cannot traverse objects, it is only suitable for array traversal.
3. for of
for...of
The statement creates a loop to iterate over an iterable object. ES6 introduced in the for...of
cycle to replace for...in
and forEach()
and support the new protocol iteration. The syntax is as follows:
for (variable of iterable) {
statement
}
This method has two parameters:
- variable: The attribute value of each iteration is assigned to the variable.
- iterable: An object with enumerable properties and iterable.
This method can get each item of the array:
let arr = [
{id:1, value:'hello'},
{id:2, value:'world'},
{id:3, value:'JavaScript'}
]
for (let item of arr) {
console.log(item);
}
// Output result:{id:1, value:'hello'} {id:2, value:'world'} {id:3, value:'JavaScript'}
Notice:
- The for of method will only traverse the properties of the current object, not the properties on the prototype chain;
- The method is suitable for of traversing the array / array data / string / map / set has iterator object set and the like;
- The for of method does not support traversal of ordinary objects, because it does not have an iterator object. If you want to traverse the properties of an object, you can use the for in method;
- You can use break, continue, return to interrupt the loop traversal;
4. filter()
filter()
The method is used to filter the array, and the elements that meet the conditions will be returned. Its parameter is a callback function, all array elements execute the function in turn, the elements whose return result is true will be returned, and if there are no elements that meet the conditions, an empty array will be returned. The syntax is as follows:
array.filter(function(currentValue,index,arr), thisValue)
The first parameter of this method is the callback function, which must be passed. It has three parameters:
- currentValue: Required. The value of the current element;
- index: optional. The index value of the current element;
- arr: Optional. The array object to which the current element belongs.
const arr = [1, 2, 3, 4, 5]
arr.filter(item => item > 2)
// Output result:[3, 4, 5]
Similarly, it also has a second parameter, which is used to bind the this variable inside the parameter function.
You can use filter()
methods to remove undefined, null, NAN and other values in the array:
let arr = [1, undefined, 2, null, 3, false, '', 4, 0]
arr.filter(Boolean)
// Output result :[1, 2, 3, 4]
Notice:
- The filter method will return a new array without changing the original array;
- The filter method will not detect empty arrays;
- The filter method is only suitable for detecting arrays.
5. some(), every()
The some() method will traverse each item in the array. As long as one element meets the condition, it will return true, and the remaining elements will not be tested again, otherwise it will return false.
The every() method traverses each item in the array, and returns true only when all elements meet the conditions. If one element in the array is not met, the entire expression returns false, and the remaining elements are not Will be tested again. The syntax is as follows:
The syntax of the two is as follows:
array.some(function(currentValue,index,arr),thisValue)
array.every(function(currentValue,index,arr), thisValue)
The first parameter of the two methods is the callback function, which must be passed. It has three parameters:
- currentValue: Required. The value of the current element;
- index: optional. The index value of the current element;
- arr: Optional. The array object to which the current element belongs.
let arr = [1, 2, 3, 4, 5]
arr.some(item => item > 4)
// Output result: true
let arr = [1, 2, 3, 4, 5]
arr.every(item => item > 0)
// Output result: true
Notice:
- Neither method will change the original array and will return a Boolean value;
- Neither method will detect empty arrays;
- Both methods are only suitable for detecting arrays.
6. reduce(), reduceRight()
The reduce() method receives a function as an accumulator, and each value in the array (from left to right) is reduced and finally calculated as a value. reduce() can be used as a higher-order function to compose the function. The syntax is as follows:
array.reduce(function(total, currentValue, currentIndex, arr), initialValue)
The reduce method will execute the callback function for each element in the array in turn, excluding the deleted or never assigned elements in the array. The callback function accepts four parameters:
- total: the value returned by the last call to the callback, or the initial value provided (initialValue);
- currentValue: the element currently being processed;
- currentIndex: the index of the current element;
- arr: The array object to which the current element belongs.
The second parameter of this method is initialValue
the initial value passed to the function (as the first parameter of the first call to callback):
let arr = [1, 2, 3, 4]
let sum = arr.reduce((prev, cur, index, arr) => {
console.log(prev, cur, index);
return prev + cur;
})
console.log(arr, sum);
Output result:
1 2 1
3 3 2
6 4 3
[1, 2, 3, 4] 10
Let's try adding an initial value:
let arr = [1, 2, 3, 4]
let sum = arr.reduce((prev, cur, index, arr) => {
console.log(prev, cur, index);
return prev + cur;
}, 5)
console.log(arr, sum);
Output result:
5 1 0
6 2 1
8 3 2
11 4 3
[1, 2, 3, 4] 15
It can be concluded that if the initial value is not provided, reduce will start the callback method at index 1, skipping the first index. If a initial value initialValue, started from the index 0
The reduceRight() method is reduce()
almost the same as its usage, except that this method traverses the array in reverse order, while the reduce()
method traverses it in positive order.
let arr = [1, 2, 3, 4]
let sum = arr.reduceRight((prev, cur, index, arr) => {
console.log(prev, cur, index);
return prev + cur;
}, 5)
console.log(arr, sum);
Output result:
5 4 3
9 3 2
12 2 1
14 1 0
[1, 2, 3, 4] 15
Notice:
- Neither method will change the original array;
- If the two methods add an initial value, it will change the original array and put this initial value at the end of the array;
- The two methods will not execute the callback function for empty arrays.
7. find(), findIndex()
find()
The method returns the value of the first element of the array determined by the function. When the element in the array returns true when the condition is tested, find()
the element that meets the condition is returned, and the execution function will not be called for the subsequent values. If there is no element that meets the conditions, undefined is returned.
findIndex()
The method returns the position (index) of the first element of the array passed in a test function that meets the conditions . When an element in the array returns true in the function condition, findIndex()
the index position of the element that meets the condition is returned, and the execution function will not be called for the subsequent values. If there is no element that meets the conditions, -1 is returned.
The syntax of the two methods is as follows:
array.find(function(currentValue, index, arr),thisValue)
array.findIndex(function(currentValue, index, arr), thisValue)
The first parameter of the two methods is the callback function, which must be passed. It has three parameters:
- currentValue: required. Current element
- index: optional. The index of the current element;
- arr: Optional. The array object to which the current element belongs.
let arr = [1, 2, 3, 4, 5]
arr.find(item => item > 2)
// Output result: 3
let arr = [1, 2, 3, 4, 5]
arr.findIndex(item => item > 2)
// Output result : 2
find()
It findIndex()
is almost the same as the two methods, except that the returned results are different:
find()
: The returned value is the first qualified value;findIndex
: What is returned is the index value of the value of the first return condition.
Notice:
- For the two methods, the function will not be executed for empty arrays;
- Whether the two methods will not change the original array.
8. keys(), values(), entries()
The three methods all return an iterative object of an array, and the content of the object is not the same:
- keys() returns the index value of the array;
- values() returns the elements of the array;
- entries() returns the key-value pairs of the array.
The syntax of the three methods is as follows:
array.keys()
array.values()
array.entries()
None of these three methods have parameters:
let arr = ["Banana", "Orange", "Apple", "Mango"];
const iterator1 = arr.keys();
const iterator2 = arr.values()
const iterator3 = arr.entries()
for (let item of iterator1) {
console.log(item);
}
// Output result: 0 1 2 3
for (let item of iterator2) {
console.log(item);
}
// Output result: Banana Orange Apple Mango
for (let item of iterator3) {
console.log(item);
}
// Output result :[0, 'Banana'] [1, 'Orange'] [2, 'Apple'] [3, 'Mango']
Summarize:
method | Whether to change the original array | Features |
---|---|---|
forEach() | no | No return value |
map() | no | Has a return value and can be chained |
for of | no | for...of traverses the properties of the object with the Iterator iterator, and returns the elements of the array and the property values of the object. It cannot traverse the ordinary obj object, turning the asynchronous loop into a synchronous loop |
filter() | no | Filter the array, return an array containing eligible elements, which can be chained |
every(), some() | no | As long as one of some() is true, it returns true; and as long as one of every() is false, it returns false. |
find(), findIndex() | no | find() returns the first value that meets the condition; findIndex() returns the index value of the first value that returns the condition |
reduce(), reduceRight() | no | reduce() operates on the positive order of the array; reduceRight() operates on the reverse of the array |
keys(), values(), entries() | no | keys() returns the index value of the array; values() returns the elements of the array; entries() returns the key-value pairs of the array. |
2) The object traversal method
1. for in
for…in
Mainly used to loop object properties. Each time the code in the loop is executed, an operation is performed on the properties of the object. The syntax is as follows:
for (var in object) {
Block of code executed
}
Two of the parameters:
- var: must. The specified variable can be an array element or an object property.
- object: Required. Specify the object to be iterated.
var obj = {a: 1, b: 2, c: 3};
for (var i in obj) {
console.log('Key name:', i);
console.log('Key value:', obj[i]);
}
Output result:
Key name: a
Key value: 1
Key name: b
Key-value: 2
Key name: c
Key-value: 3
Notice:
- The for in method not only traverses all enumerable properties of the current object, but also traverses the properties on the prototype chain.
2. Object.keys(), Object.values(), Object.entries()
These three methods are used to traverse the object, it will return an array composed of the enumerable properties of the given object (excluding inherited and Symbol properties), the sequence of the array elements and the return when the object is traversed in a normal loop The order is the same, and the values returned by the three elements are as follows:
- Object.keys(): returns an array containing object keys;
- Object.values(): returns an array containing object key values;
- Object.entries(): Returns an array containing object keys and key values.
let obj = {
id: 1,
name: 'hello',
age: 18
};
console.log(Object.keys(obj)); // Output result: ['id', 'name', 'age']
console.log(Object.values(obj)); // Output result: [1, 'hello', 18]
console.log(Object.entries(obj)); // Output result: [['id', 1], ['name', 'hello'], ['age', 18]
Notice
- The values in the array returned by the Object.keys() method are all strings, which means that key values that are not strings will be converted into strings.
- The attribute values in the result array are all enumerable attributes of the object itself , excluding inherited attributes.
3. Object.getOwnPropertyNames()
Object.getOwnPropertyNames()
The method is Object.keys()
similar. It also accepts an object as a parameter and returns an array containing all the attribute names of the object itself. But it can return non-enumerable properties.
let a = ['Hello', 'World'];
Object.keys(a) // ["0", "1"]
Object.getOwnPropertyNames(a) // ["0", "1", "length"]
Both of these methods can be used to count the number of attributes in an object:
var obj = { 0: "a", 1: "b", 2: "c"};
Object.getOwnPropertyNames(obj) // ["0", "1", "2"]
Object.keys(obj).length // 3
Object.getOwnPropertyNames(obj).length // 3
4. Object.getOwnPropertySymbols()
Object.getOwnPropertySymbols()
The method returns an array of Symbol properties of the object itself, excluding string properties:
let obj = {a: 1}
// Add a non-enumerable Symbol property to the object
Object.defineProperties(obj, {
[Symbol('baz')]: {
value: 'Symbol baz',
enumerable: false
}
})
// Add an enumerable Symbol property to the object
obj[Symbol('foo')] = 'Symbol foo'
Object.getOwnPropertySymbols(obj).forEach((key) => {
console.log(obj[key])
})
// Output :Symbol baz Symbol foo
5. Reflect.ownKeys()
Reflect.ownKeys() returns an array containing all the properties of the object itself. It is similar to Object.keys(), Object.keys() returns the attribute key, but does not include non-enumerable attributes, while Reflect.ownKeys() returns all attribute keys:
var obj = {
a: 1,
b: 2
}
Object.defineProperty(obj, 'method', {
value: function () {
alert("Non enumerable property")
},
enumerable: false
})
console.log(Object.keys(obj))
// ["a", "b"]
console.log(Reflect.ownKeys(obj))
// ["a", "b", "method"]
Notice:
- Object.keys(): equivalent to returning an array of object attributes;
- Reflect.ownKeys(): equivalent
Object.getOwnPropertyNames(obj).concat(Object.getOwnPropertySymbols(obj)
.
Summarize:
Object method | Traverse basic attributes | Traverse the prototype chain | Traverse non-enumerable properties | Traverse Symbol |
---|---|---|---|---|
for in | Yes | Yes | no | no |
Object.keys() | Yes | no | no | no |
Object.getOwnPropertyNames() | Yes | no | Yes | no |
Object.getOwnPropertySymbols() | no | no | Yes | Yes |
Reflect.ownKeys() | Yes | no | Yes | Yes |
3) Other traversal methods
1. for
The for loop should be the most common way of looping. It consists of three expressions, which are declaring loop variables, judging loop conditions, and updating loop variables. These three expressions are separated by semicolons. Temporary variables can be used to cache the length of the array to avoid repeated acquisition of the length of the array. When the array is large, the optimization effect will be more obvious.
const arr = [1,2,3,4,5]
for(let i = 0, len = arr.length; i < len; i++ ){
console.log(arr[i])
}
During execution, the execution conditions will be judged before execution. The for loop can be used to traverse arrays, strings, arrays, DOM nodes, etc. The original array can be changed.
2. while
while
The end conditions in the loop can be of various types, but they will eventually be converted to Boolean values. The conversion rules are as follows.
- Boolean: true is true, false is false;
- String: An empty string is false, and all non-empty strings are true;
- Number: 0 is false, non-zero numbers are true;
- null/Undefined/NaN: all false;
- Object: All true.
let num = 1;
while (num < 10){
console.log(num);
num ++;
}
while
And for
, we are the first judge, and then executed. As long as the specified condition is true, the loop can continue to execute the code.
3. do / while
This method will be executed first and then judged. Even if the initial conditions are not established, the do/while
loop will be executed at least once.
let num = 10;
do
{
console.log(num);
num--;
}
while(num >= 0);
console.log(num); //-1
It is not recommended to use do / while to traverse the array.
4. for await of
for await...of
The method is called an asynchronous iterator, and the method is mainly used to traverse asynchronous objects. It is the method introduced in ES2018.
for await...of
The statement creates an iterative loop on asynchronous or synchronous iterable objects, including String, Array, array-like, Map, Set, and custom asynchronous or synchronous iterable objects. This statement can only be async function
in the use of:
function Gen (time) {
return new Promise((resolve,reject) => {
setTimeout(function () {
resolve(time)
},time)
})
}
async function test () {
let arr = [Gen(2000),Gen(100),Gen(3000)]
for await (let item of arr) {
console.log(Date.now(),item)
}
}
test()
Output result:
What's Your Reaction?
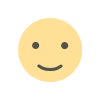
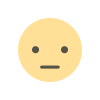




