10 React techniques that every beginner should know
React is a popular JavaScript library for building user interfaces. There are several techniques that every beginner should know in order to be effective in using React. These techniques include the use of state, props, hooks, components, JSX, conditional rendering, event handling, lifecycle methods, context, and refs.
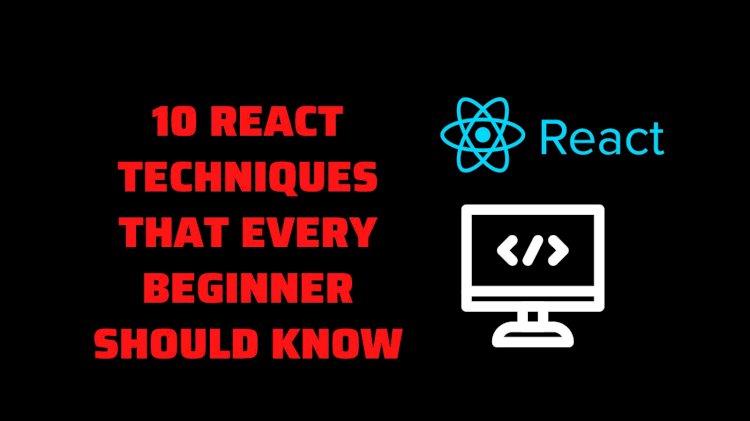
React is a popular JavaScript library for building user interfaces. There are several techniques that every beginner should know in order to be effective in using React. These techniques include the use of state, props, hooks, components, JSX, conditional rendering, event handling, lifecycle methods, context, and refs.
Here are 10 React techniques that every beginner should know:
-
Virtual DOM: The Virtual DOM is one of the core concepts of React. It allows React to update the UI efficiently by only rendering the elements that have changed. Understanding how the Virtual DOM works is crucial for building efficient and performant React applications.
ExampleComponent
receivesdata
as a prop and renders a list of items using themap
method. The Virtual DOM will keep track of the changes to the list and only update the elements that have changed, improving the performance of the application.
-
Components: Components are the building blocks of a React application. They allow you to split your UI into reusable pieces, making it easier to manage and maintain your code. You should learn how to create and use components, as well as understand the difference between stateful and stateless components.
In this example,Welcome
is a stateless component that receivesname
as a prop and renders a greeting.App
is the parent component that uses theWelcome
component twice to render greetings for two different names. -
Props: Props are properties that you can pass from a parent component to a child component. This is a key concept for building dynamic and reusable components, as it allows you to easily customize the behavior of your components based on your needs.
In this example,
App
passesuser.name
as a prop to theWelcome
component. TheWelcome
component uses this prop to render a personalized greeting. -
State: State is an object that holds data that can change over time. In React, you should keep your state in the component that needs to use it, and only update it using the
setState
method. This helps to ensure that your components are always in sync with the latest data.
In this example,Counter
uses theuseState
hook to manage its state. The state is represented by thecount
variable, which is updated using thesetCount
function. The component renders a button that increments the count when clicked. -
Conditional rendering: Conditional rendering is the process of showing or hiding elements based on certain conditions. You can use the ternary operator or the
&&
operator to conditionally render elements in your components.
In this example, theUser
component conditionally renders a greeting or a message based on the value of theisLoggedIn
prop. IfisLoggedIn
istrue
, the component will render a greeting. IfisLoggedIn
isfalse
, the component will render a message asking the user to log in. -
Event handling: Event handling is the process of responding to user interactions, such as clicks or form submissions. You can handle events in React by passing a callback function as a prop to a component or by using the
useState
hook to update your component's state.
In this example, the component uses an event handler to update its state in response to a user action. The event handlerhandleClick
is triggered when the button is clicked and updates the value ofmessage
using thesetMessage
function. -
Forms: Forms are a common part of many web applications, and React provides a set of tools for building and managing forms. You should learn how to use the
form
andinput
elements, as well as understand how to handle form submissions and validate form inputs.
In this example, the component uses a controlled component to manage the state of an input field. The value of the input field is controlled by theemail
state variable, and thehandleChange
function updates the value ofemail
in response to changes in the input field. The component also implements a submit handler to demonstrate how the state can be used to process form submissions. -
Lifecycle methods: Lifecycle methods are methods that are called at specific points during the lifecycle of a component. You should understand the purpose of each lifecycle method and when to use them, such as
componentDidMount
for performing setup logic after a component has mounted.
In this example, the state is lifted up from theCounter
component to its parent component,App
. The parent component manages theinitialCount
state and passes it down as a prop to theCounter
component. This allows the parent component to control the behavior of multiple instances of theCounter
component. -
Hooks: Hooks are a new feature in React that allow you to add state and other React features to functional components. They are a powerful tool for managing component state and improving code reusability.
In this example, theThemeContext
is created using thecreateContext
function. TheThemeToggler
component provides the current theme value using theThemeContext.Provider
component. TheThemeButton
component uses theuseContext
hook to access the current theme value from the context. This allows the theme value to be shared between components without the need for props drilling. -
Performance optimization: Performance optimization is an important aspect of building React applications. You should understand how to use the React Developer Tools to identify performance bottlenecks and how to use techniques such as memoization and lazy loading to improve performance.
In this example, theuseRef
hook is used to create a reference to the input element. The reference is stored in theinputEl
variable, which can be used to access the input element directly. ThehandleClick
function uses thefocus
method to set the focus on the input element when the button is clicked. This demonstrates how refs can be used to access DOM elements directly from React components.
These are just a few of the key concepts that every beginner should know when learning React. By mastering these techniques, you'll be well on your way to building dynamic and powerful React applications.
What's Your Reaction?
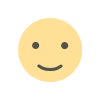
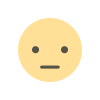




