Getting Started with PyQt for GUI Development
PyQt, a powerful library for creating graphical user interfaces (GUIs) in Python, has gained immense popularity due to its versatility and ease of use. Whether you're a beginner or an experienced developer, mastering the most used PyQt code snippets is essential for efficient GUI development. Let's delve into the key aspects of PyQt coding and explore the most utilized code snippets that can elevate your Python GUI interface.
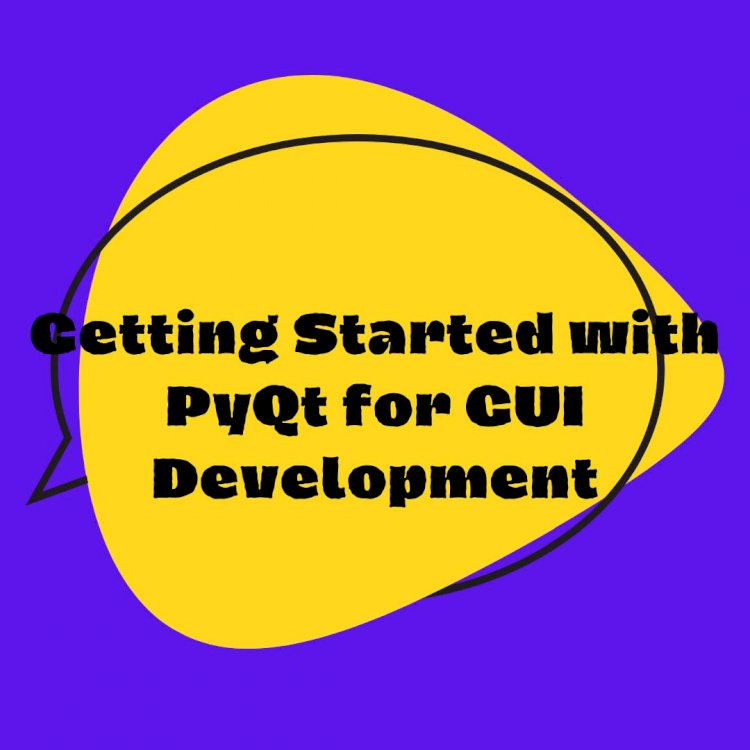
PyQt, a powerful library for creating graphical user interfaces (GUIs) in Python, has gained immense popularity due to its versatility and ease of use. Whether you're a beginner or an experienced developer, mastering the most used PyQt code snippets is essential for efficient GUI development. Let's delve into the key aspects of PyQt coding and explore the most utilized code snippets that can elevate your Python GUI interface.
Introduction
PyQt, a set of Python bindings for the Qt application framework, enables developers to create cross-platform applications with native-looking GUIs. It seamlessly integrates with Python, offering a wide array of functionalities for GUI development.
Getting Started with PyQt for GUI Development
To kickstart your PyQt journey, install the library using the following command:
pip install PyQt5
Ensure you have Python installed, and you're ready to embark on your GUI development adventure.
Basic PyQt Code Structure
The fundamental structure of a PyQt application involves creating a QApplication and a QMainWindow. Here's a basic template:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
app = QApplication(sys.argv)
window = QMainWindow()
window.show()
sys.exit(app.exec_())
This code sets up a basic PyQt application window, and you can build upon this foundation for more complex interfaces.
Creating Widgets in PyQt
Widgets are the building blocks of a PyQt GUI. Let's look at how to create some commonly used widgets:
Buttons:
from PyQt5.QtWidgets import QPushButton
button = QPushButton("Click me")
button.clicked.connect(self.on_button_click)
This code creates a clickable button and connects it to a function (on_button_click
) that gets executed when the button is pressed.
Labels:
from PyQt5.QtWidgets import QLabel
label = QLabel("Hello, PyQt!")
Adding a label to your GUI is as simple as this. Labels are useful for displaying text or information.
Text Boxes:
from PyQt5.QtWidgets import QLineEdit
text_box = QLineEdit()
Creating a text box allows users to input text. You can access the entered text programmatically for further processing.
Handling Events in PyQt
PyQt follows an event-driven programming paradigm. Let's handle a button click event:
def on_button_click(self):
print("Button clicked!")
By defining a function like this, you respond to user interactions effectively.
Layout Management in PyQt
Efficient layout management ensures your GUI looks organized and user-friendly. Utilize layout managers like QVBoxLayout, QHBoxLayout, or QGridLayout for optimal results.
Vertical Layout:
from PyQt5.QtWidgets import QVBoxLayout
layout = QVBoxLayout()
layout.addWidget(button)
layout.addWidget(label)
This organizes widgets vertically, making your interface aesthetically pleasing.
Using Stylesheets in PyQt
Customizing the appearance of your GUI is a breeze with stylesheets:
app.setStyleSheet("QPushButton { background-color: #3498db; color: #ffffff; }")
This example changes the button's background color to a soothing blue and the text color to white.
Integration of Icons and Images
Enhance your GUI by integrating icons and images:
label.setPixmap(QtGui.QPixmap('path/to/image.png'))
Replace 'path/to/image.png' with the actual path to your image file.
Working with Menus and Toolbars
Menus and toolbars provide a structured way for users to interact with your application. Here's a basic example:
from PyQt5.QtWidgets import QMenu, QMenuBar, QToolBar
menu_bar = self.menuBar()
file_menu = menu_bar.addMenu('File')
# Add an action to the menu
exit_action = QAction('Exit', self)
exit_action.triggered.connect(self.close)
file_menu.addAction(exit_action)
This snippet creates a menu bar with a 'File' menu and an 'Exit' action.
Data Input and Output in PyQt
Incorporate input fields and handle user input effortlessly:
input_text = text_box.text()
Access the entered text using text_box.text()
and process it as needed.
Advanced PyQt Features
Take your GUI development to the next level with advanced features like drag-and-drop functionality:
button.setAcceptDrops(True)
button.dragEnterEvent = self.dragEnterEvent
button.dropEvent = self.dropEvent
These functions enable drag-and-drop support for your button.
Debugging PyQt Code
Debugging PyQt applications can be simplified by using the built-in Python debugger. Insert the following line where you want to set a breakpoint:
import pdb; pdb.set_trace()
This allows you to inspect variables and step through your code.
Optimizing PyQt Code for Performance
Ensure your PyQt code runs smoothly by optimizing it:
# Use efficient algorithms for data manipulation
# Avoid unnecessary widget updates
# Optimize resource-intensive operations
Adopting these practices enhances the performance of your PyQt application.
Integration with Databases in PyQt
Connect your PyQt application with databases seamlessly:
import sqlite3
# Connect to a SQLite database
conn = sqlite3.connect('example.db')
Replace 'example.db' with the name of your database file.
Testing and Deployment
Before deploying your PyQt application, thorough testing is crucial. Utilize tools like unittest
to ensure your code is robust. Once testing is complete, deploy your application using packaging tools like PyInstaller or cx_Freeze.
Conclusion
Mastering the most used PyQt code snippets is pivotal for creating dynamic and user-friendly GUIs in Python. From basic widget creation to advanced features, PyQt empowers developers to build cross-platform applications efficiently. As you delve into the world of PyQt, experiment with these code snippets, customize them to suit your needs, and watch your GUI come to life.
FAQs
-
What is PyQt?
- PyQt is a set of Python bindings for the Qt application framework, allowing developers to create cross-platform applications with native-looking GUIs.
-
How can I install PyQt?
- You can install PyQt using the command
pip install PyQt5
after ensuring you have Python installed.
- You can install PyQt using the command
-
What are widgets in PyQt?
- Widgets are the building blocks of a PyQt GUI, such as buttons, labels, and text boxes.
-
How can I handle events in PyQt?
- Events in PyQt are handled by connecting signals (like button clicks) to functions using the
connect
method.
- Events in PyQt are handled by connecting signals (like button clicks) to functions using the
-
What is the significance of layout management in PyQt?
- Layout management ensures a well-organized and user-friendly GUI by using managers like QVBoxLayout, QHBoxLayout, or QGridLayout.
What's Your Reaction?
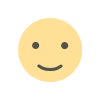
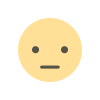




